namespace
mathnamespace for math
Functions
-
template<typename T1, typename T2>auto min(T1 const& x, T2 const& y) -> auto constexpr
- SpaceHub min.
-
template<typename T1, typename T2>auto max(T1 const& x, T2 const& y) -> auto constexpr
- SpaceHub max.
-
template<typename T>auto abs(const T& x) -> T constexpr
- SpaceHub abs.
-
template<typename T>auto in_range(T low, T x, T high) -> T constexpr
- Truncate a number in a range.
-
template<typename T>auto step(T x) -> T constexpr
- Step function.
-
template<typename T>auto sign(T x) -> T constexpr
- Sign function.
-
template<typename T>auto iseq(T x, T y) -> bool
-
template<typename Fun>auto root_bisection(Fun f, decltype(f(0)) low, decltype(f(0)) high) -> auto
- find root use bisection method
-
template<typename Fun>auto root_newton(Fun f) -> decltype(std::declval<Fun>()(0))
- Find root with Newton method.
Variables
-
template<typename T>T epsilon_v constexpr
- Min numerical limit(epsilon) of a floating point type/.
-
template<typename T>T max_value_v constexpr
- Max value of a specific type.
-
template<typename T>T big_value_v constexpr
- A big value of a specific type.
Function documentation
template<typename T1, typename T2>
auto hub:: math:: min(T1 const& x,
T2 const& y) constexpr
SpaceHub min.
Template parameters | |
---|---|
T1 | Type of the first argument. |
T2 | Type of the second argument. |
Parameters | |
x in | The first argument. |
y in | The second argument. |
Returns | T2 The min of x, y; |
template<typename T1, typename T2>
auto hub:: math:: max(T1 const& x,
T2 const& y) constexpr
SpaceHub max.
Template parameters | |
---|---|
T1 | Type of the first argument. |
T2 | Type of the second argument. |
Parameters | |
x in | The first argument. |
y in | The second argument. |
Returns | T2 The max of x, y; |
template<typename T>
T hub:: math:: abs(const T& x) constexpr
SpaceHub abs.
Template parameters | |
---|---|
T | Type of the argument. |
Parameters | |
x in | The input argument. |
Returns | T The absolute value. |
template<typename T>
T hub:: math:: in_range(T low,
T x,
T high) constexpr
Truncate a number in a range.
Template parameters | |
---|---|
T | Type of the truncated variable. |
Parameters | |
low in | The lower limit. |
x in | The variable need to be truncated. |
high in | The higher limit. |
Returns | T The truncated value. |
template<typename T>
T hub:: math:: step(T x) constexpr
Step function.
Template parameters | |
---|---|
T | Type of the x, y. |
Parameters | |
x in | |
Returns | constexpr T y |
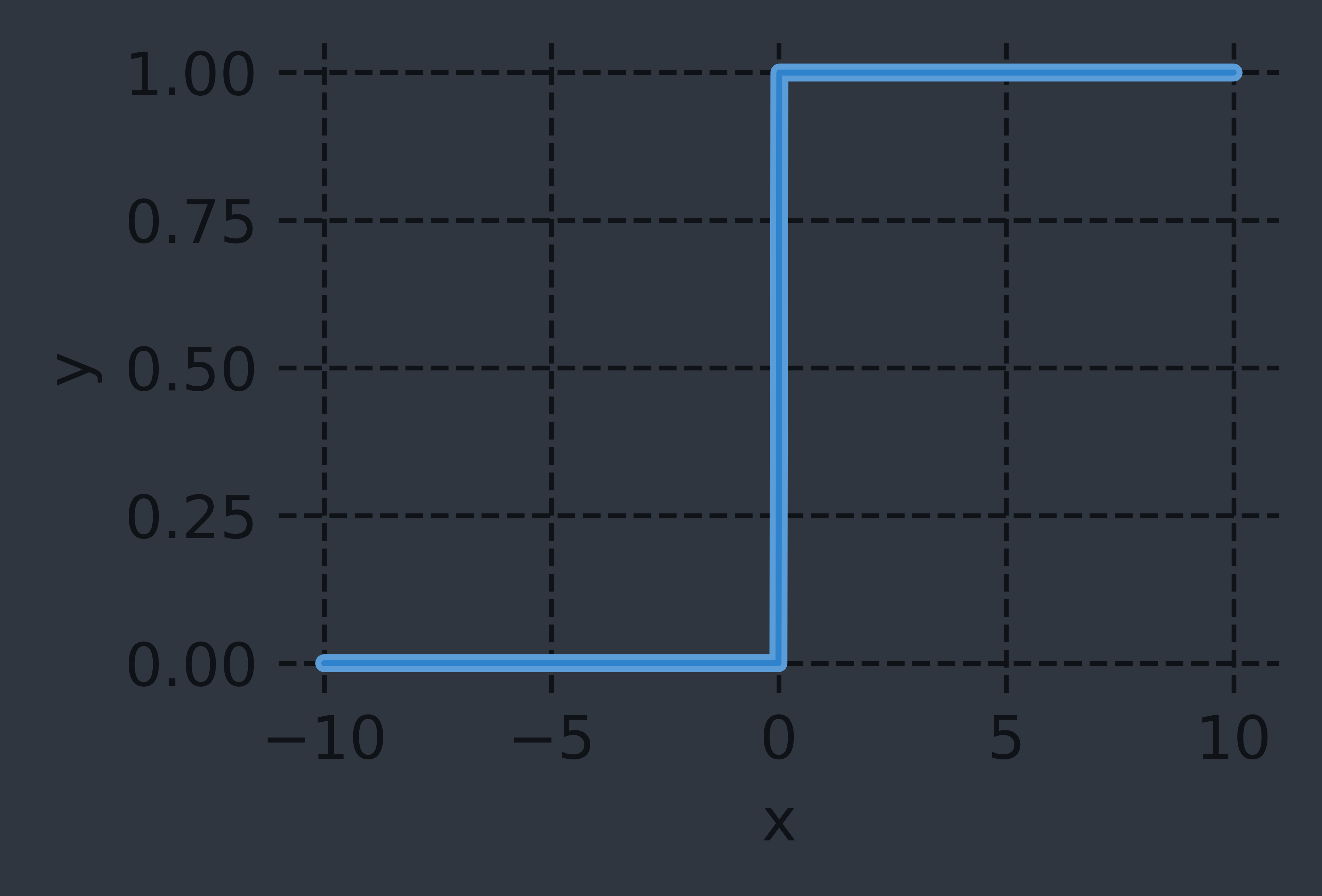
template<typename T>
T hub:: math:: sign(T x) constexpr
Sign function.
Template parameters | |
---|---|
T | Type of x, y |
Parameters | |
x in | |
Returns | constexpr T y |
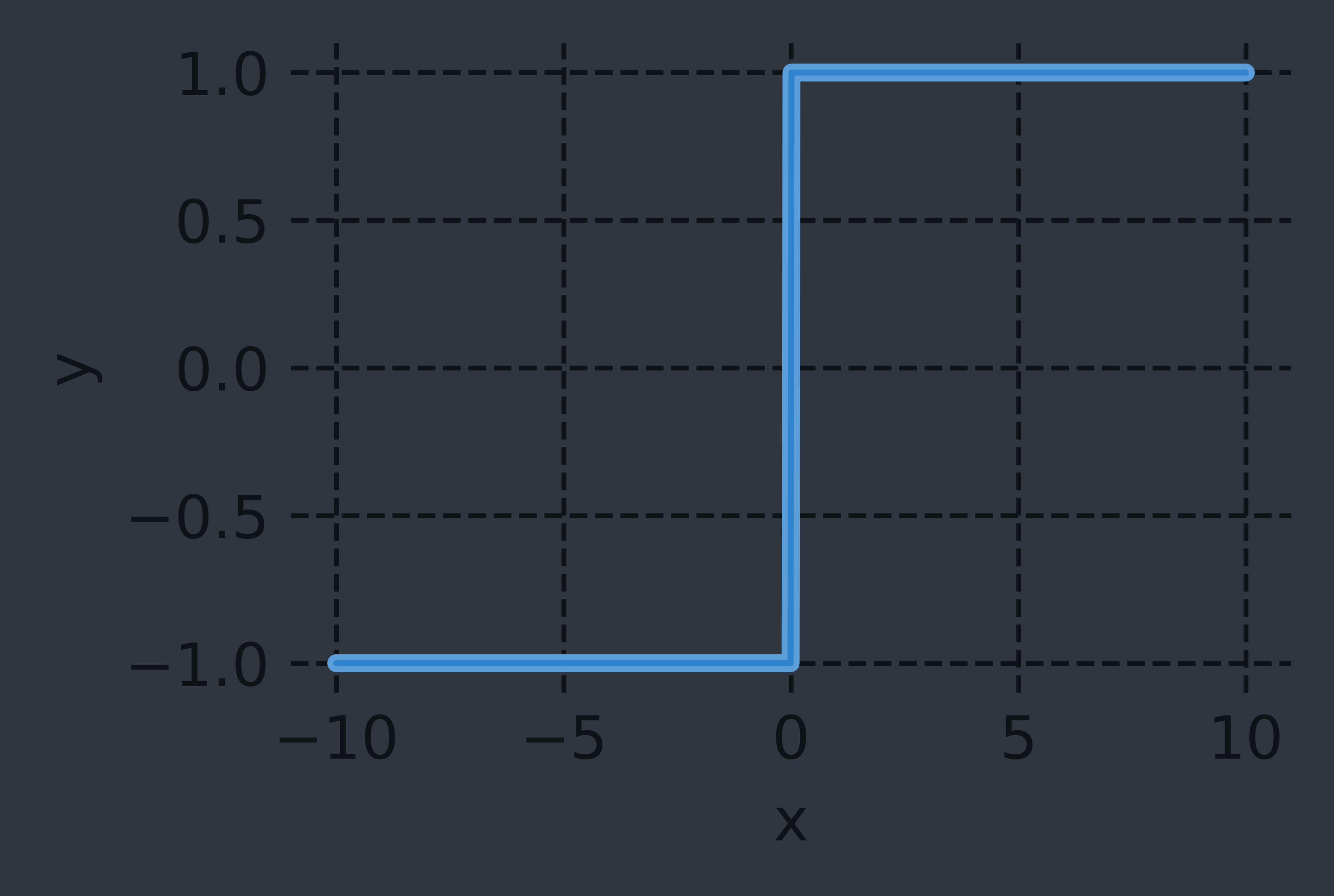
template<typename T>
bool hub:: math:: iseq(T x,
T y)
Template parameters | |
---|---|
T | |
Parameters | |
x | |
y | |
Returns | true |
template<typename Fun>
auto hub:: math:: root_bisection(Fun f,
decltype(f(0)) low,
decltype(f(0)) high)
find root use bisection method
Template parameters | |
---|---|
Fun | Type of Callable object. |
Parameters | |
f | Callable object |
low | Lower limit of root range |
high | Upper limit of root range |
Returns | decltype(f(0)) The root. |
template<typename Fun>
decltype(std::declval<Fun>()(0)) hub:: math:: root_newton(Fun f)
Find root with Newton method.
Template parameters | |
---|---|
Fun | Type of callable object. |
Parameters | |
f | Callable object. |
Returns | decltype(std::declval<Fun>()(0)) The root. |
Variable documentation
template<typename T>
T hub:: math:: epsilon_v constexpr
Min numerical limit(epsilon) of a floating point type/.
Template parameters | |
---|---|
T |
template<typename T>
T hub:: math:: max_value_v constexpr
Max value of a specific type.
Template parameters | |
---|---|
T |
template<typename T>
T hub:: math:: big_value_v constexpr
A big value of a specific type.
Template parameters | |
---|---|
T |
0.1 max_value_v